Using Boost Library with Eclipse
Introduction
The purpose of this blog is to show how to use the boost library in Eclipse CDT plug-in with Cygwin and MinGW compiler.
About Boost Library:
Boost is an Internet community dedicated to building and reviewing C++ libraries. The initial members of Boost were C++ Standards Committee members who wanted to create a forum for developing libraries that might some day become part of the C++ Standard. Boost emphasize libraries that work well with the C++ Standard Library. Boost libraries are intended to be widely useful, and usable across a broad spectrum of applications. The Boost license encourages both commercial and non-commercial use. Boost works on almost any modern operating system, including UNIX and Windows variants. Follow the Getting Started Guide to download and install Boost. Popular Linux and Unix distributions such as Fedora, Debian, and NetBSD include pre-built Boost packages.
Eclipse CDT:
The CDT Project provides a fully functional C and C++ Integrated Development Environment based on the Eclipse platform. Features include: support for project creation and managed build for various toolchains, standard make build, source navigation, various source knowledge tools, such as type hierarchy, call graph, include browser, macro definition browser, code editor with syntax highlighting, folding and hyperlink navigation, source code refactoring and code generation, visual debugging tools, including memory, registers, and disassembly viewers.
( click here to download eclipse for C++)
Cygwin:
Cygwin is a Linux-like environment for Windows. It consists of two parts:
- A DLL (cygwin1.dll) which acts as a Linux API emulation layer providing substantial Linux API functionality.
- A collection of tools which provide Linux look and feel.
The Cygwin DLL currently works with all recent, commercially released x86 32 bit and 64 bit versions of Windows, with the exception of Windows CE.
MinGW:
- MinGW, a contraction of “Minimalist GNU for Windows”, is a minimalist development environment for native Microsoft Windows applications.
- MinGW provides a complete Open Source programming tool set which is suitable for the development of native MS-Windows applications, and which do not depend on any 3rd-party C-Runtime DLLs (only the Microsoft C runtime, MSVCRT).
Downloading and Installing Cyginwin:
Note: click here to download cygwin
Follow the following steps to install cygwin,
- Run the Cyginwin setup.
- Select install from Internet
- Set the root directory as C:\cygwin
- Set the local directory for the installation files
- Configure the internet settings, Direction connection or Proxy settings.
- Select the mirror site
- Select the core components or allow the default selection and give next.
Download and Install MinGW compiler:
Click here to download MinGW compiler
Download the MinGW compiler and accept the default settings and install the MinGW compiler
Make sure to select the GCC compler
Download boost Source and bjam tool:
Click here to download the boost source and bjam tool.
Installing Boost library:
- Add the path of the cygwin in environment variable
- Add the path of minGW compiler in environment variable
- Extract the Eclipse in C:\Eclipse directory
- Extract the boost package in C:\Program Files\boost\
- Extract the bjam tool in C:\Program Files\boost\boostxxx.\
- Go to the command prompt give the following command
C:\Program Files\boost\boost_1_44_0>bjam --toolset=gcc --build-type=complete
Note: build process will take lot of time to finish. Wait patiently.
Using Boost lib in Eclipse
- Open Eclipse->File -> New -> C++ project
- Empty project and accept other default options
- Right click the project and add new Source file ( shown in the screen shot )
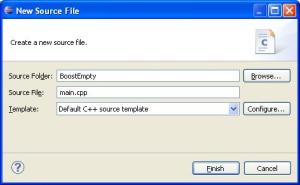
- Select the project Properties
- Select the path and Symbol’s under C/C++ General category.
- Select GNU C and click add button
Now you can enter the boost path or explore the path using File System button. it show like as follows.
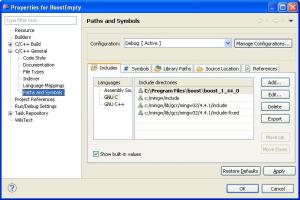
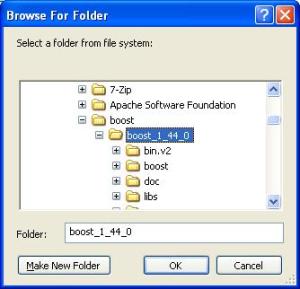
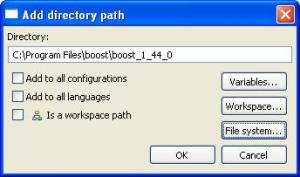
Repeat the same process for GNU C++
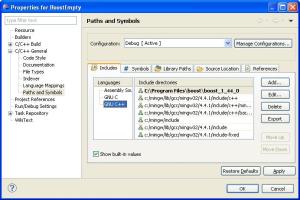
Adding the code:
Add the following code in main.cpp file
#include <iostream>
#include <boost/foreach.hpp>
using namespace std;
int main() {
cout<<"Boost Demo"<<endl;
int a[10];
for(int i=0;i<10;i++)
{
a[i]=i+10;
}
BOOST_FOREACH(int k, a)
{
cout<<"\t"<<k;
}
return 0;
}
Build and Run the Project:
Click project-> Build Project
Click Run Command to run the project
See the output in Console tab